Dive into the fabulous world of computer systems! Understanding what a computer system is and how it runs your programs can help you to design code that runs efficiently and that can make the best use of the power of the underlying system. In this book, we take you on a journey through computer systems. You will learn how your program written in a high-level programming language (we use C) executes on a computer. You will learn how program instructions translate into binary and how circuits execute their binary encoding. You will learn how an operating system manages programs running on the system. You will learn how to write programs that can make use of multicore computers. Throughout, you will learn how to evaluate the systems costs associated with program code and how to design programs to run efficiently.
컴퓨터 시스템의 멋진 세계로 뛰어들어 보세요! 컴퓨터 시스템이 무엇인지, 그리고 프로그램을 실행하는 방식을 이해하면 효율적으로 작동하고 기본 시스템의 성능을 최대한 활용할 수 있는 코드를 설계하는 데 도움이 됩니다. 이 책에서는 여러분을 컴퓨터 시스템의 여정으로 안내합니다. 고급 프로그래밍 언어(여기서는 C 사용)로 작성된 프로그램이 컴퓨터에서 실행되는 방식을 배우게 됩니다. 프로그램 명령어가 어떻게 이진수로 변환되고, 회로가 이진 인코딩을 실행하는지 알게 될 것입니다. 또한 운영 체제가 시스템에서 실행 중인 프로그램을 어떻게 관리하는지도 배울 것입니다. 멀티코어 컴퓨터를 활용할 수 있는 프로그램 작성법도 익히게 됩니다. 전반적으로, 프로그램 코드와 관련된 시스템 비용을 평가하는 방법과 효율적으로 실행되도록 프로그램을 설계하는 방법을 배우게 될 것입니다.
What Is a Computer System?
A computer system combines the computer hardware and special system software that together make the computer usable by users and programs. Specifically, a computer system has the following components
- Input/output (IO) ports enable the computer to take information from its environment and display it back to the user in some meaningful way.
- A central processing unit (CPU) runs instructions and computes data and memory addresses.
- Random access memory (RAM) stores the data and instructions of running programs. The data and instructions in RAM are typically lost when the computer system loses power.
- Secondary storage devices like hard disks store programs and data even when power is not actively being provided to the computer.
- An operating system (OS) software layer lies between the hardware of the computer and the software that a user runs on the computer. The OS implements programming abstractions and interfaces that enable users to easily run and interact with programs on the system. It also manages the underlying hardware resources and controls how and when programs execute. The OS implements abstractions, policies, and mechanisms to ensure that multiple programs can simultaneously run on the system in an efficient, protected, and seamless manner.
The first four of these define the computer hardware component of a computer system. The last item (the operating system) represents the main software part of the computer system. There may be additional software layers on top of an OS that provide other interfaces to users of the system (e.g., libraries). However, the OS is the core system software that we focus on in this book.
We focus specifically on computer systems that have the following qualities:
- They are general purpose, meaning that their function is not tailored to any specific application.
- They are reprogrammable, meaning that they support running a different program without modifying the computer hardware or system software.
To this end, many devices that may "compute" in some form do not fall into the category of a computer system. Calculators, for example, typically have a processor, limited amounts of memory, and I/O capability. However, calculators typically do not have an operating system (advanced graphing calculators like the TI-89 are a notable exception to this rule), do not have secondary storage, and are not general purpose.
Another example that bears mentioning is the microcontroller, a type of integrated circuit that has many of the same capabilities as a computer. Microcontrollers are often embedded in other devices (such as toys, medical devices, cars, and appliances), where they control a specific automatic function. Although microcontrollers are general purpose, reprogrammable, contain a processor, internal memory, secondary storage, and are I/O capable, they lack an operating system. A microcontroller is designed to boot and run a single specific program until it loses power. For this reason, a microcontroller does not fit our definition of a computer system.
컴퓨터 시스템이란?
컴퓨터 시스템은 하드웨어와 소프트웨어가 통합되어 다양한 작업을 수행할 수 있도록 설계된 장치입니다. 사용자 입력을 받아 데이터를 처리하고, 정보를 저장하며, 의미 있는 출력을 제공합니다. 아래는 컴퓨터 시스템의 구성 요소와 특징에 대한 상세 설명입니다.
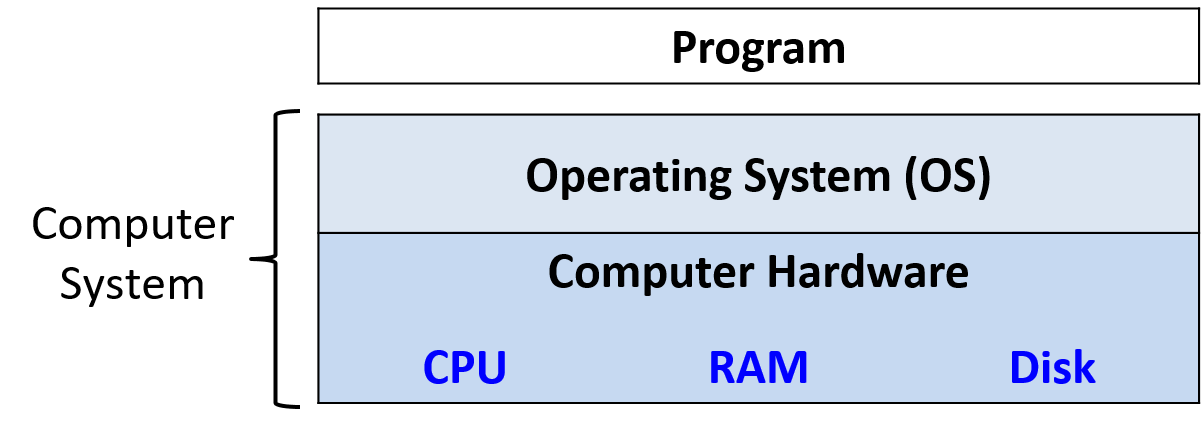
컴퓨터 시스템의 구성 요소
- 하드웨어 구성 요소:
- 입출력(I/O) 장치: 시스템과 상호작용할 수 있도록 합니다.
- 입력 장치: 키보드, 마우스 등 데이터를 입력하는 장치.
- 출력 장치: 모니터, 프린터 등 결과를 출력하는 장치.
- 중앙처리장치(CPU): 컴퓨터의 "두뇌"로 불리며, 명령어를 실행하고 계산을 수행하며 데이터 흐름을 관리합니다.
- CPU의 하위 구성 요소:
- 산술논리장치(ALU): 수학적 계산 및 논리 연산 수행.
- 제어장치: CPU 내에서 작업을 지시하고 조정.
- CPU의 하위 구성 요소:
- 메모리:
- 랜덤 액세스 메모리(RAM): 실행 중인 프로그램의 데이터와 명령어를 일시적으로 저장. RAM의 데이터는 전원이 꺼지면 사라짐.
- 보조 저장 장치: 하드 드라이브나 SSD와 같은 장치는 전원이 꺼져도 데이터를 영구적으로 저장.
- 메인보드(마더보드): 모든 하드웨어 구성 요소를 연결하고 통신을 가능하게 함.
- 입출력(I/O) 장치: 시스템과 상호작용할 수 있도록 합니다.
- 소프트웨어 구성 요소:
- 운영 체제(OS): 하드웨어와 응용 소프트웨어 사이에서 중개 역할을 합니다. 자원을 관리하고 사용자 인터페이스를 제공하며 여러 프로그램이 효율적이고 안전하게 실행될 수 있도록 보장합니다.
- 응용 소프트웨어: 워드 프로세서나 웹 브라우저와 같이 특정 작업을 수행하는 프로그램.
컴퓨터 시스템의 특징
- 범용성:
- 계산기와 같은 특수 목적 장치와 달리, 컴퓨터 시스템은 다양한 프로그램을 실행할 수 있는 다목적성을 가집니다.
- 재프로그래밍 가능성:
- 컴퓨터는 실행할 소프트웨어나 명령어를 변경함으로써 다양한 작업을 수행할 수 있습니다.
- 속도와 효율성:
- 대량의 데이터를 빠르고 정확하게 처리합니다.
- 자동화:
- 한 번 프로그래밍되면 인간의 개입 없이도 작동할 수 있습니다.
- 저장 능력:
- 단기(RAM) 및 장기(보조 저장 장치) 데이터를 대량으로 저장할 수 있습니다.
컴퓨터 시스템으로 간주되지 않는 것들
일부 장치는 계산 작업을 수행할 수 있지만, 이 정의에 따라 컴퓨터 시스템으로 간주되지 않습니다:
- 계산기: 산술 연산을 수행하지만 운영 체제, 보조 저장 장치 및 범용성을 갖추고 있지 않음.
- 마이크로컨트롤러: 특정 작업을 수행하도록 설계된 임베디드 시스템으로 운영 체제가 없습니다. 전원이 꺼질 때까지 단일 프로그램만 실행하도록 설계되어 있으며, 주로 전자제품(전자레인지, 자동차 등)에 내장됩니다.
결론적으로, 컴퓨터 시스템은 하드웨어와 소프트웨어가 결합되어 사용자가 다양한 작업을 효율적으로 수행할 수 있도록 돕는 동적인 장치입니다. 그 범용성과 재프로그래밍 가능성, 그리고 복잡한 작업을 관리하는 능력은 계산기나 마이크로컨트롤러와 같은 단순한 계산 장치와 구별되는 특징입니다.
What Do Modern Computer Systems Look Like?
Now that we have established what a computer system is (and isn’t), let’s discuss what computer systems typically look like. Figure 2 depicts two types of computer hardware systems (excluding peripherals): a desktop computer (left) and a laptop computer (right). A U.S. quarter on each device gives the reader an idea of the size of each unit.
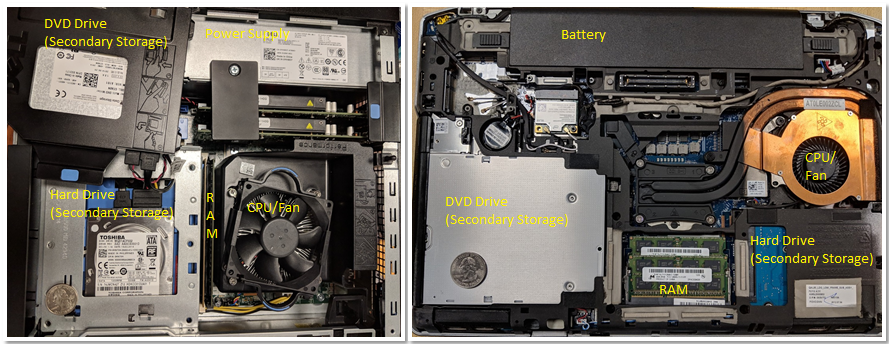
Figure 2. Common computer systems: a desktop (left) and a laptop (right) computer
Notice that both contain the same hardware components, though some of the components may have a smaller form factor or be more compact. The DVD/CD bay of the desktop was moved to the side to show the hard drive underneath — the two units are stacked on top of each other. A dedicated power supply helps provide the d
esktop power.
In contrast, the laptop is flatter and more compact (note that the quarter in this picture appears a bit bigger). The laptop has a battery and its components tend to be smaller. In both the desktop and the laptop, the CPU is obscured by a heavyweight CPU fan, which helps keep the CPU at a reasonable operating temperature. If the components overheat, they can become permanently damaged. Both units have dual inline memory modules (DIMM) for their RAM units. Notice that laptop memory modules are significantly smaller than desktop modules.
In terms of weight and power consumption, desktop computers typically consume 100 - 400 W of power and typically weigh anywhere from 5 to 20 pounds. A laptop typically consumes 50 - 100 W of power and uses an external charger to supplement the battery as needed.
The trend in computer hardware design is toward smaller and more compact devices. Figure 3 depicts a Raspberry Pi single-board computer. A single-board computer (SBC) is a device in which the entirety of the computer is printed on a single circuit board.
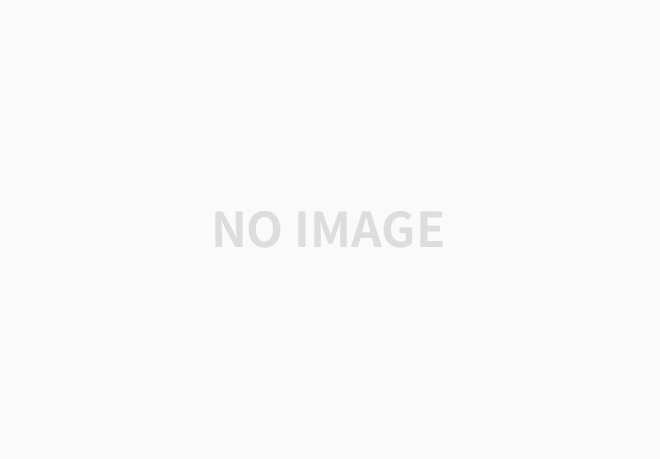
The Raspberry Pi SBC contains a system-on-a-chip (SoC) processor with integrated RAM and CPU, which encompasses much of the laptop and desktop hardware shown in Figure 2. Unlike laptop and desktop systems, the Raspberry Pi is roughly the size of a credit card, weighs 1.5 ounces (about a slice of bread), and consumes about 5 W of power. The SoC technology found on the Raspberry Pi is also commonly found in smartphones. In fact, the smartphone is another example of a computer system!
Lastly, all of the aforementioned computer systems (Raspberry Pi and smartphones included) have multicore processors. In other words, their CPUs are capable of executing multiple programs simultaneously. We refer to this simultaneous execution as parallel execution. Basic multicore programming is covered in Chapter 14 of this book.
All of these different types of computer hardware systems can run one or more general purpose operating systems, such as macOS, Windows, or Unix. A general-purpose operating system manages the underlying computer hardware and provides an interface for users to run any program on the computer. Together these different types of computer hardware running different general-purpose operating systems make up a computer system.
현대 컴퓨터 시스템은 다양한 형태와 크기로 제공되며, 데스크톱, 노트북, 싱글보드 컴퓨터(SBC), 스마트폰 등 여러 종류가 있습니다. 이들은 각각의 목적과 사용 환경에 맞게 설계되어 있으며, 공통적으로 중앙처리장치(CPU), 메모리, 저장 장치, 전원 공급 장치 등을 포함합니다
데스크톱 컴퓨터
- 구성 요소: 데스크톱은 일반적으로 CPU, RAM(DIMM 모듈), 하드 드라이브 또는 SSD, 전원 공급 장치가 포함된 물리적 유닛과 모니터, 키보드, 마우스 등의 주변 기기로 구성됩니다.
- 특징:
- 크기가 크고 무게가 5~20파운드(약 2.3~9kg)로 무겁습니다.
- 전력 소비량은 약 100~400W입니다.
- 업그레이드와 수리가 용이하며 고성능 작업(예: 그래픽 디자인, 게임)에 적합합니다.
- 냉각 시스템: CPU는 고성능 팬으로 냉각되며, 과열 시 손상이 발생할 수 있습니다
노트북 컴퓨터
- 구성 요소: 노트북은 데스크톱과 동일한 하드웨어를 가지고 있지만 더 작고 통합된 형태로 설계되었습니다. RAM 모듈은 소형화된 SODIMM을 사용합니다.
- 특징:
- 크기가 작고 무게가 가벼우며(약 1~3kg), 휴대성이 뛰어납니다.
- 배터리를 포함하며 전력 소비량은 약 50~100W입니다.
- 대부분의 부품이 내장되어 있어 업그레이드와 수리가 비교적 어렵습니다
싱글보드 컴퓨터 (Raspberry Pi 등)
- 구성 요소: Raspberry Pi와 같은 SBC는 단일 회로 보드에 CPU, RAM, 저장 장치 및 I/O 포트를 통합한 시스템입니다.
- 특징:
- 크기가 신용카드 정도로 작고 무게는 약 1.5온스(43g)입니다.
- 전력 소비량이 매우 낮아 약 5W만 필요합니다.
- SoC(System-on-a-Chip) 기술을 사용하여 스마트폰과 유사한 구조를 가집니다
스마트폰
- 스마트폰은 SoC 프로세서를 기반으로 하며, SBC와 유사한 구조를 가지고 있지만 터치스크린 디스플레이와 다양한 센서가 추가되어 있습니다. 이들은 고도로 통합된 컴퓨터 시스템으로 간주됩니다.
공통 특징
- 멀티코어 프로세서: 모든 현대 컴퓨터 시스템은 멀티코어 CPU를 사용하여 병렬 처리가 가능하며 여러 프로그램을 동시에 실행할 수 있습니다.
- 운영 체제: macOS, Windows, Linux 등 범용 운영 체제를 실행하여 사용자 인터페이스를 제공하고 하드웨어를 관리합니다.
- 소형화 및 에너지 효율성: 기술 발전으로 인해 컴퓨터는 점점 더 작아지고 에너지 효율성이 높아지고 있습니다.
현대 컴퓨터 시스템은 다양한 형태로 제공되지만, 공통적으로 강력한 처리 능력과 유연성을 갖추고 있어 개인용부터 산업용까지 폭넓게 사용되고 있습니다.
https://computerinfobits.com/parts-of-computer-and-their-functions/
Parts Of A Computer And Their Functions Explained
One of the first things to learn is the parts of a computer and their functions. It is vital to gain an insight into the internal workings of a computer.
computerinfobits.com
https://spca.education/pc-components/
https://slideplayer.com/slide/12329610/
CHAPTER 11: Modern Computer Systems - ppt download
Basic Personal Computer System Copyright 2013 John Wiley & Sons, Inc.
slideplayer.com
$ uname -a
Linux Fawkes 4.4.0-171-generic #200-Ubuntu SMP Tue Dec 3 11:04:55 UTC 2019
x86_64 x86_64 x86_64 GNU/Linux
- The kernel name of the system (in this case Linux)
- The hostname of the machine (e.g., Fawkes)
- The kernel release (e.g., 4.4.0-171-generic)
- The kernel version (e.g., #200-Ubuntu SMP Tue Dec 3 11:04:55 UTC 2019)
- The machine hardware (e.g., x86_64)
- The type of processor (e.g., x86_64)
- The hardware platform (e.g., x86_64)
- The operating system name (e.g., GNU/Linux)
https://diveintosystems.org/book/introduction.html#FigLayeredComponents
Dive Into Systems
Dive into the fabulous world of computer systems! Understanding what a computer system is and how it runs your programs can help you to design code that runs efficiently and that can make the best use of the power of the underlying system. In this book, we
diveintosystems.org
'컴퓨터 > deep dive into systems' 카테고리의 다른 글
1. By the C, by the C, by the Beautiful C-1.1. Getting Started Programming in C (1) | 2025.01.26 |
---|